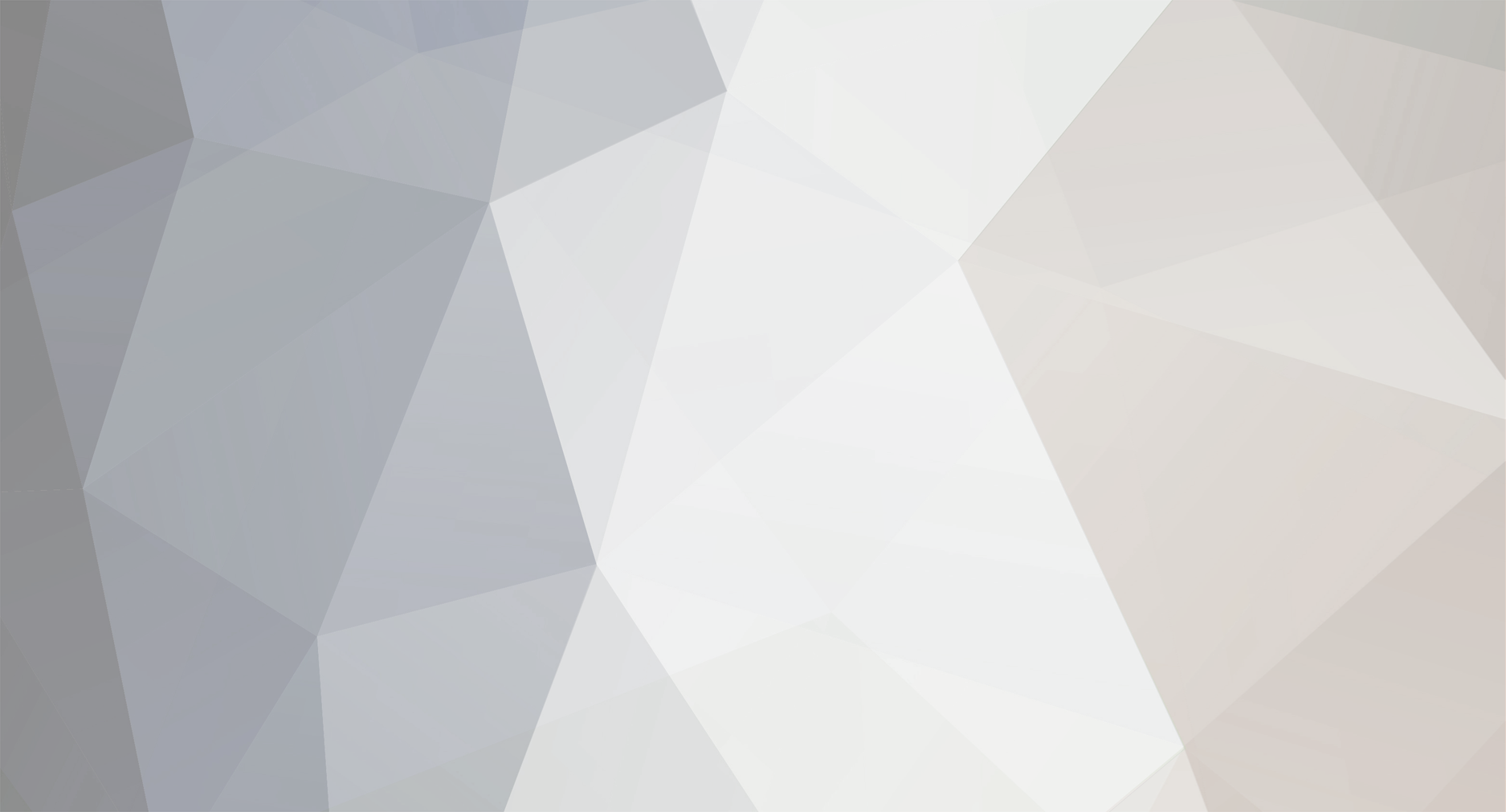
oxysoft
Members-
Posts
25 -
Joined
-
Last visited
Profile Information
-
Gender
Not Telling
Contact Methods
-
Twitter
oxysofts
oxysoft's Achievements
-
Hey thanks, it seems to work! I wrote these two functions to make it easier to create the layers and objects in the proper rendering order. It could be useful to someone! createLayer(name) { if (this.map.getLayer(name) === null) return; let layer = this.map.createLayer(name); this.layers.push(layer); } createObjects(name, gid, key, index = -1, group = this.game.world) { if (this.map.objects[name] == undefined) return; let sprite; for (let i = 0, len = this.map.objects[name].length; i < len; i++) { if (this.map.objects[name][i].gid == gid || gid == -1) { sprite = new Phaser.Sprite(this.game, this.map.objects[name][i].x + this._x, this.map.objects[name][i].y, key, index == -1 ? this.map.objects[name][i].gid - 1 : index); sprite.name = this.map.objects[name][i].name; sprite.visible = true; // this.map.objects[name][i].visible sprite.autoCull = false; sprite.exists = true; sprite.y -= sprite.height; group.add(sprite); for (let prop in this.map.objects[name][i].properties) { group.set(sprite, property, this.map.objects[name][i].properties[prop], false, false, 0, true); } } } }Usage // waterthis.createLayer('water');// terrain 1this.createLayer('terrain');// objects 1this.createObjects('objects', -1, 'tileset');// terrain 2this.createLayer('terrain2');// objects 2this.createObjects('objects2', -1, 'tileset');And here is the result! https://gfycat.com/AmusingAgreeableGrub
-
I am making an "infinite runner" type of game where the map is seemingly infinite. The maps will not be procedurally generated but an arrengement of segments instead. I have devised a SegmentManager type of structure in my code which would push new segments to the end as the player's actor reach the end of the buffered segments. It seeks the segments in batches of 5 since most segments are around 10 tiles worth of 16 pixels in width each. It would be enough to cover up a nice distance until more segments have to be buffered. Segments that are already passed and are no longer needed are discarded and possibly reused for later buffering. Each segments of course consist of tiled maps that can all be put together back to back and will look good at all time. This implementation has worked fine in my previous codebase which was put together using LibGDX and Java. I have ported the code to es6 because I want to learn more about Phaser since it seems like a very nice framework to hack up games really quickly, but I seem to have hit a wall. So far, it seems nearly impossible to have multiple tilemaps and move them around the scene. So is there a correct way to do this? It seems like a bad design to me and I should be able to have multiple maps that can be moved about, and that are all independant of each other. I have read that certain workarounds will break collisions as well, which is undesirable since I will at some point want collisions to happen on certain layers of these segments
-
Haha yeah, that is indeed very true. I think the teddy bear pair programming is more popular under the name of rubber duck debugging!
-
Nevermind, It should have been Tilemap and not TILEMAP. Odd that it didn't result in any error though, setting up a difficult situation to debug. Kinda weird how I noticed immediately after posting
-
I thought it could have been my tiled json that was improper or whatever but I tried using the Desert.json along with the tileset from the phaser examples and it's the same result Picture: This is the code used to create the map and the ground layer var map = this.game.add.tilemap('desert');map.addTilesetImage('desert', 'tiles');var layer = map.createLayer(0);Note that createLayer('Ground')has no effect and yields the following error message in the console Tilemap.createLayer: Invalid layer ID given: nullSo I logged to the console the name of the layer which is supposed to be Ground according to the json file and phaser examples and it came up as layer for some reasons Furthermore, the width of the map is always equal to 2 despite being set to 40 in the json file. Logging to the console more properties from the map seems to reveal that they are all set to default values. So is it failing to read the json or what? This is the statement used to load it in the preload function this.game.load.tilemap('desert', 'assets/desert.json', null, Phaser.TILEMAP.TILED_JSON);Thanks
-
I am trying to use TypeScript with phaser and I am getting the following errors Error 7 Class 'TileSprite' incorrectly extends base class 'TilingSprite': Types of property 'destroy' are incompatible: Type '(destroyChildren: boolean) => void' is not assignable to type '() => void'. c:\Users\oxysoft\documents\visual studio 2013\Projects\flint-game\flint-game\phaser.d.ts 4270 11 flint-game I have added Phaser.js, Phaser.js.min, Phaser.d.ts and Pixi.d.ts and it is not working Thanks
-
While this is a sound theory, it turns out that turning off the text doesn't fix the issue Have a look at the updated webgl version: http://pokesharp.com/maple3/webgl/ It still falls down to ~48 fps The fps text still updates but was optimized to be once per second only. (which shouldn't cause any problem) I tried commenting out all of the physics stuff, leaving only var sprite = entityGroup.create(x, 100, 'sprites', '' + j + '.png');and the problem persisted. As one would expect, commenting out that line indeed brings the fps back to 60 when holding the mouse button
-
Oh another thing that would be nice in Phaser 3 is a couple of effects/filters that come prepackaged and that could be enabled per sprite with ease (i.e glow, drop shadow) It should be easy to customize the parameters of the effects too (glow color, glow strength, etc.)
-
I have encountered what seems to be a weird fluke that causes WebGL to be much slower than canvas. I will let you see for yourself webgl: http://pokesharp.com/maple3/webgl/ canvas: http://pokesharp.com/maple3/canvas/ With webgl, the fps seems to drop by about 10 while the game is spawning entities. If you release the mouse however, it goes back to 60. Meanwhile, canvas sails at a smooth 60 constantly. Here is the code. I am using P2 and the text stuff could probably be stripped as it is unlikely to be the source of the issue var game = new Phaser.Game("100%", "100%", Phaser.WEBGL, "phaser", { preload: preload, create: create, update: update });var max = 1000;var titleText, fpsText, entitiesText;var entityGroup;var mouseDown = false; function preload() { game.time.advancedTiming = true; game.load.atlasXML('sprites', 'assets/sprites.png', 'assets/sprites.xml'); game.load.physics('body', 'assets/body.json'); game.load.audio('pain', 'assets/sounds.mp3');} function create() { game.physics.startSystem(Phaser.Physics.P2JS); game.physics.p2.restitution = 0.2; game.physics.p2.gravity.y = 300; entityGroup = game.add.group(); var style = { font: '30px Arial', fill: '#ff0044', border: '#ffffff', align: 'center'}; titleText = game.add.text(0, 0, 'Click to feel good', style) titleText.anchor.set(0.5, 0.5); style = { font: '16px Arial', fill: '#333333', align: 'center'}; fpsText = game.add.text(0, 0, 'fps: ', style); entitiesText = game.add.text(0, 0, 'entities: ', style); updateTexts(); game.input.onDown.add(function() { mouseDown = true; }); game.input.onUp.add(function() { mouseDown = false; });} function updateTexts() { titleText.x = game.width / 2 - 5; titleText.y = game.height / 2; titleText.rotation = 0; titleText.text = 'Click to acquire feels'; if (mouseDown) { titleText.x += Math.floor(Math.random() * 100) - 50; titleText.y += Math.floor(Math.random() * 100) - 50; titleText.rotation = (Math.floor(Math.random() * 50) - 25) * Math.PI/180; titleText.text = 'IT FEELS PRETTY GOOD'; } fpsText.text = 'fps: '; fpsText.x = 5; fpsText.y = 5; fpsText.text += game.time.fps; entitiesText.text = 'entities: '; entitiesText.x = 5; entitiesText.y = fpsText.y + fpsText.height; entitiesText.text += entityGroup.length;} var tick = 0; function update() { tick++; updateTexts(); if (tick % 5 == 0 && mouseDown) { var j = game.rnd.integerInRange(0, max - 1); var x = game.width / 2; var sprite = entityGroup.create(x, 100, 'sprites', '' + j + '.png'); game.physics.p2.enable(sprite, false); sprite.body.damping = 0.5; var lspeed = 800; var hspeed = 700; sprite.body.velocity.x = Math.random() < 0.5 ? game.rnd.integerInRange(-lspeed, -hspeed) : game.rnd.integerInRange(lspeed, hspeed); sprite.body.velocity.y = -300; sprite.body.allowSleep = true; } if (mouseDown) { var v = Math.floor(Math.random() * 4).toString(16); game.stage.backgroundColor = '#' + v + v + v; } else { game.stage.backgroundColor = 0; }}I'd be very interested in knowing why this happens and how I can avoid it
-
An easy way to scale the game up WITHOUT antialiasing. This is such a pain to do right now and we have to resort to weird chacks etc. Best case scenario: I would set my game to any size, i.e 100x100 and set the scale to 2. The resulting html5 canvas would be 200x200. The scale would then be easily changeable in the future if I want my game to be rendered at any other scale. This is important for games with scaled pixel art and it's a pain to export our art already prescaled (and not good for bandwidth/size and such!)
-
oxysoft reacted to a post in a topic: The Phaser 3 Wishlist Thread :)
-
Would it be too late to add a offset point to the camera? That would make camera shake so much easier to add into our games
-
Add a crouch sprite to your character's spritesheet and adjust the sprite's bounding box with body.setSize(...) whenever the entity is crouching
-
The sleep deprivation of this weekend must have really gotten to me! It's fixed now
-
Dreaming of Revenge Unfinished by a fluke; Had I had like 2 or 3 more hours, (which I would have had if I hadn't been so inexperienced to Phaser at the beginning of this Ludum Dare) it would probably have been 100% complete Does anyone know what are the rules regarding modifying the game's static information? (Entity spawn positions in a map, an entity's navigation mesh, etc) I would assume it's not allowed since only gamebreaking bugs are okay to fix Or maybe adding sound effects and music post Ludum Dare? At least, the game has very pretty and incredibly polished graphics, thanks to my invaluable teammate
-
I want to add screen shake to my game but can't find a good way to do it. I was hoping the camera object would have an offset point that I could have set to whatever I want without having to touch the camera's position but that was not the case. After that, I checked in World and because it is a group, I tried setting its fixedToCamera to true and then use cameraOffset but, much to my deception, did not work either The only *solution* I found by searching online was to use an outdated plugin or set the World's bounds, which I rather not do because the bounds is used to keep sprites inside and I do not want to have to deal with any bug that could arise from it What are my options? I really wish there was a camera offset, that would be very useful